JWT in Cookies
Highly recommended using JWT in cookies, if your frontend interacts with the backend, your frontend may be storing JWT in the browser localStorage or sessionStorage. There is nothing wrong with this, but if you have any sort of XSS vulnerability on your site, an attacker will be able to trivially steal your tokens. If you want some additional security on your site, you can save your JWT in an httponly cookies. Which keeps javascript cannot be able to access the cookies.
Here is a basic example of how to store JWT in cookies:
Note
You can also create cookies or unset cookies when returning a Response
directly in your code.
To do that, you can create a response then set the response in set cookies or unset cookies
...
response = JSONResponse(content={"msg":"Successfully login"})
# Set the JWT and CSRF double submit cookies in the response
Authorize.set_access_cookies(access_token,response)
Authorize.set_refresh_cookies(refresh_token,response)
return response
from fastapi import FastAPI, HTTPException, Depends, Request
from fastapi.responses import JSONResponse
from fastapi_jwt_auth import AuthJWT
from fastapi_jwt_auth.exceptions import AuthJWTException
from pydantic import BaseModel
"""
Note: This is just a basic example how to enable cookies.
This is vulnerable to CSRF attacks, and should not be used this example.
"""
app = FastAPI()
class User(BaseModel):
username: str
password: str
class Settings(BaseModel):
authjwt_secret_key: str = "secret"
# Configure application to store and get JWT from cookies
authjwt_token_location: set = {"cookies"}
# Disable CSRF Protection for this example. default is True
authjwt_cookie_csrf_protect: bool = False
@AuthJWT.load_config
def get_config():
return Settings()
@app.exception_handler(AuthJWTException)
def authjwt_exception_handler(request: Request, exc: AuthJWTException):
return JSONResponse(
status_code=exc.status_code,
content={"detail": exc.message}
)
@app.post('/login')
def login(user: User, Authorize: AuthJWT = Depends()):
if user.username != "test" or user.password != "test":
raise HTTPException(status_code=401,detail="Bad username or password")
# Create the tokens and passing to set_access_cookies or set_refresh_cookies
access_token = Authorize.create_access_token(subject=user.username)
refresh_token = Authorize.create_refresh_token(subject=user.username)
# Set the JWT cookies in the response
Authorize.set_access_cookies(access_token)
Authorize.set_refresh_cookies(refresh_token)
return {"msg":"Successfully login"}
@app.post('/refresh')
def refresh(Authorize: AuthJWT = Depends()):
Authorize.jwt_refresh_token_required()
current_user = Authorize.get_jwt_subject()
new_access_token = Authorize.create_access_token(subject=current_user)
# Set the JWT cookies in the response
Authorize.set_access_cookies(new_access_token)
return {"msg":"The token has been refresh"}
@app.delete('/logout')
def logout(Authorize: AuthJWT = Depends()):
"""
Because the JWT are stored in an httponly cookie now, we cannot
log the user out by simply deleting the cookies in the frontend.
We need the backend to send us a response to delete the cookies.
"""
Authorize.jwt_required()
Authorize.unset_jwt_cookies()
return {"msg":"Successfully logout"}
@app.get('/protected')
def protected(Authorize: AuthJWT = Depends()):
"""
We do not need to make any changes to our protected endpoints. They
will all still function the exact same as they do when sending the
JWT in via a headers instead of a cookies
"""
Authorize.jwt_required()
current_user = Authorize.get_jwt_subject()
return {"user": current_user}
This isn't the full story. However now we can keep our cookies from being stolen via XSS attacks, but session cookies vulnerable to CSRF attacks. To combat CSRF attacks we are going to use a technique called double submit cookie pattern. Double submitting cookies is defined as sending a random value in both a cookie and as a request parameter, with the server verifying if the cookie value and request value are equal.
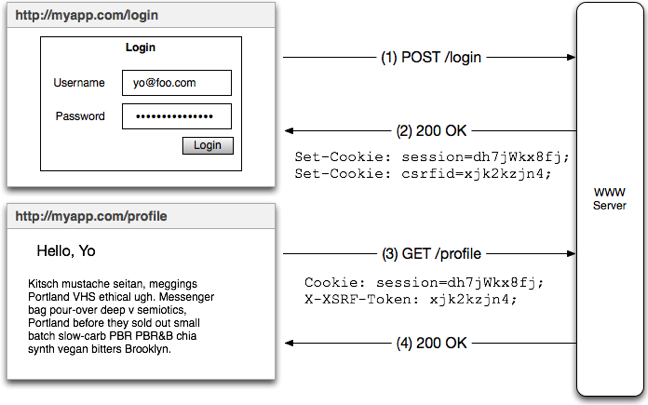
This tokens is saved in a cookie with httponly set to True, so it cannot be accessed via javascript. We will then create a secondary cookie that contains an only random string, but has httponly set to False so that it can be accessed via javascript running on your website.
Now in order to access a protected endpoint, you will need to add a custom header that contains the random string in it, and if that header doesn’t exist or it doesn’t match the string that is stored in the JWT, the requester will be kicked out as unauthorized.
To break this down, if an attacker attempts to perform a CSRF attack they will send the JWT (via cookie) to protected endpoint, but without the random string in the request headers, they won't be able to access the endpoint. They cannot access the random string unless they can run javascript on your website likely via an XSS attack, and if they are able to perform an XSS attack, they will not be able to steal the actual access and refresh JWT, as javascript is still not able to access those httponly cookies.
No system is safe. If an attacker can perform an XSS attack they can still access protected endpoints from people who visit your site. However, it is better than if they were able to steal the access and refresh tokens from local/session storage, and use them whenever they wanted.
Here is an example of using cookies with CSRF protection:
from fastapi import FastAPI, HTTPException, Depends, Request
from fastapi.responses import JSONResponse
from fastapi_jwt_auth import AuthJWT
from fastapi_jwt_auth.exceptions import AuthJWTException
from pydantic import BaseModel
"""
By default, the CRSF cookies will be called csrf_access_token and
csrf_refresh_token, and in protected endpoints we will look
for the CSRF token in the 'X-CSRF-Token' headers. only certain
methods should define CSRF token in headers default is ('POST','PUT','PATCH','DELETE')
"""
app = FastAPI()
class User(BaseModel):
username: str
password: str
class Settings(BaseModel):
authjwt_secret_key: str = "secret"
# Configure application to store and get JWT from cookies
authjwt_token_location: set = {"cookies"}
# Only allow JWT cookies to be sent over https
authjwt_cookie_secure: bool = False
# Enable csrf double submit protection. default is True
authjwt_cookie_csrf_protect: bool = True
# Change to 'lax' in production to make your website more secure from CSRF Attacks, default is None
# authjwt_cookie_samesite: str = 'lax'
@AuthJWT.load_config
def get_config():
return Settings()
@app.exception_handler(AuthJWTException)
def authjwt_exception_handler(request: Request, exc: AuthJWTException):
return JSONResponse(
status_code=exc.status_code,
content={"detail": exc.message}
)
@app.post('/login')
def login(user: User, Authorize: AuthJWT = Depends()):
"""
With authjwt_cookie_csrf_protect set to True, set_access_cookies() and
set_refresh_cookies() will now also set the non-httponly CSRF cookies
"""
if user.username != "test" or user.password != "test":
raise HTTPException(status_code=401,detail="Bad username or password")
# Create the tokens and passing to set_access_cookies or set_refresh_cookies
access_token = Authorize.create_access_token(subject=user.username)
refresh_token = Authorize.create_refresh_token(subject=user.username)
# Set the JWT and CSRF double submit cookies in the response
Authorize.set_access_cookies(access_token)
Authorize.set_refresh_cookies(refresh_token)
return {"msg":"Successfully login"}
@app.post('/refresh')
def refresh(Authorize: AuthJWT = Depends()):
Authorize.jwt_refresh_token_required()
current_user = Authorize.get_jwt_subject()
new_access_token = Authorize.create_access_token(subject=current_user)
# Set the JWT and CSRF double submit cookies in the response
Authorize.set_access_cookies(new_access_token)
return {"msg":"The token has been refresh"}
@app.delete('/logout')
def logout(Authorize: AuthJWT = Depends()):
"""
Because the JWT are stored in an httponly cookie now, we cannot
log the user out by simply deleting the cookie in the frontend.
We need the backend to send us a response to delete the cookies.
"""
Authorize.jwt_required()
Authorize.unset_jwt_cookies()
return {"msg":"Successfully logout"}
@app.get('/protected')
def protected(Authorize: AuthJWT = Depends()):
Authorize.jwt_required()
current_user = Authorize.get_jwt_subject()
return {"user": current_user}